In this 5-10 minute introduction to the Visual Studio integrated development environment (IDE), you'll create a simple Node.js web application.
Prerequisites
You must have Visual Studio installed and the Node.js development workload.
If you haven't already installed Visual Studio 2019, go to the Visual Studio downloads page to install it for free.
If you haven't already installed Visual Studio 2017, go to the Visual Studio downloads page to install it for free.
If you need to install the workload but already have Visual Studio, go to Tools > Get Tools and Features.., which opens the Visual Studio Installer. Choose the Node.js development workload, then choose Modify.
You must have the Node.js runtime installed.
If you don't have it installed, we recommend you install the LTS version from the Node.js website for best compatibility with outside frameworks and libraries. Node.js is built for 32-bit and 64-bit architectures. The Node.js tools in Visual Studio, included in the Node.js workload, support both versions. Only one is required and the Node.js installer only supports one being installed at a time.
In general, Visual Studio automatically detects the installed Node.js runtime. If it does not detect an installed runtime, you can configure your project to reference the installed runtime in the properties page (after you create a project, right-click the project node, choose Properties, and set the Node.exe path). You can use a global installation of Node.js or you can specify the path to a local interpreter in each of your Node.js projects.
Create a project
NW.js (previously known as node-webkit) lets you call all Node.js modules directly from DOM and enables a new way of writing applications with all Web technologies. New way of writing native applications using web technologies: HTML5, CSS3, and WebGL. Full support for the features in browser. Hyperledger Fabric offers a number of SDKs to support developing smart contracts (chaincode) in various programming languages. There are two other smart contract SDKs available for Java, and Go, in addition to this Node.js SDK. With Node.js you can build complex single-page apps, chat rooms, data streaming apps, browser games and even do hardware programming. Find out what else you can use Node.js for, as well as the cases when this run-time environment is not a fit. Download Node.js for most platforms. Download the IBM i Node.js SDK. If you want to use IBM i with Node.js, use our RPM, which bundles our Node.js SDK with other supported open source packages. The installation is simple and you’ll be sure that Node.js will work well in your IBM i environment. Download Node.js for IBM i.
First, you'll create an Node.js web application project.
If you don't have the Node.js runtime already installed, install the LTS version from the Node.js website.
For more information, see the prerequisites.
Open Visual Studio.
Create a new project.
Press Esc to close the start window. Type Ctrl + Q to open the search box, type Node.js, then choose Create new Blank Node.js Web application project (JavaScript). In the dialog box that appears, choose Create.
From the top menu bar, choose File > New > Project. In the left pane of the New Project dialog box, expand JavaScript, then choose Node.js. In the middle pane, choose Blank Node.js Web application, then choose OK.
If you don't see the Blank Node.js Web application project template, you must add the Node.js development workload. For detailed instructions, see the Prerequisites.
Visual Studio creates and the new solution and opens the project. server.js opens in the editor in the left pane.
Explore the IDE
Take a look at Solution Explorer in the right pane.
Highlighted in bold is your project, using the name you gave in the New Project dialog box. On disk, this project is represented by a .njsproj file in your project folder.
At the top level is a solution, which by default has the same name as your project. A solution, represented by a .sln file on disk, is a container for one or more related projects.
The npm node shows any installed npm packages. You can right-click the npm node to search for and install npm packages using a dialog box.
If you want to install npm packages or Node.js commands from a command prompt, right-click the project node and choose Open Command Prompt Here.
In the server.js file in the editor (left pane), choose
http.createServer
and then press F12 or choose Go To Definition from the context (right-click) menu. This command takes you to the definition of thecreateServer
function in index.d.ts.Got back to server.js, then put your cursor at the end of the string in this line of code,
res.end('Hello Worldn');
, and modify it so that it looks like this:res.end('Hello Worldn' + res.connection.
Where you type
connection.
, IntelliSense provides options to auto-complete the code entry.Choose localPort, and then type
);
to complete the statement so that it looks like this:res.end('Hello Worldn' + res.connection.localPort);
Run the application
Press Ctrl+F5 (or Debug > Start Without Debugging) to run the application. The app opens in a browser.
In the browser window, you will see 'Hello World' plus the local port number.
Close the web browser.
Congratulations on completing this Quickstart in which you got started with the Visual Studio IDE and Node.js. If you'd like to delve deeper into its capabilities, continue with a tutorial in the Tutorials section of the table of contents.
Next steps
Raspberry Pi is a small, multi-use computer.
With Node.js you can do amazing things with your Raspberry Pi.
What is the Raspberry Pi?
The Raspberry Pi is a small, affordable, and amazingly capable, credit card size computer.
It is developed by the Raspberry Pi Foundation, and it might be the most versatile tech ever created.
Creator Eben Upton's goal was to create a low-cost device that would improve programming skills and hardware understanding.
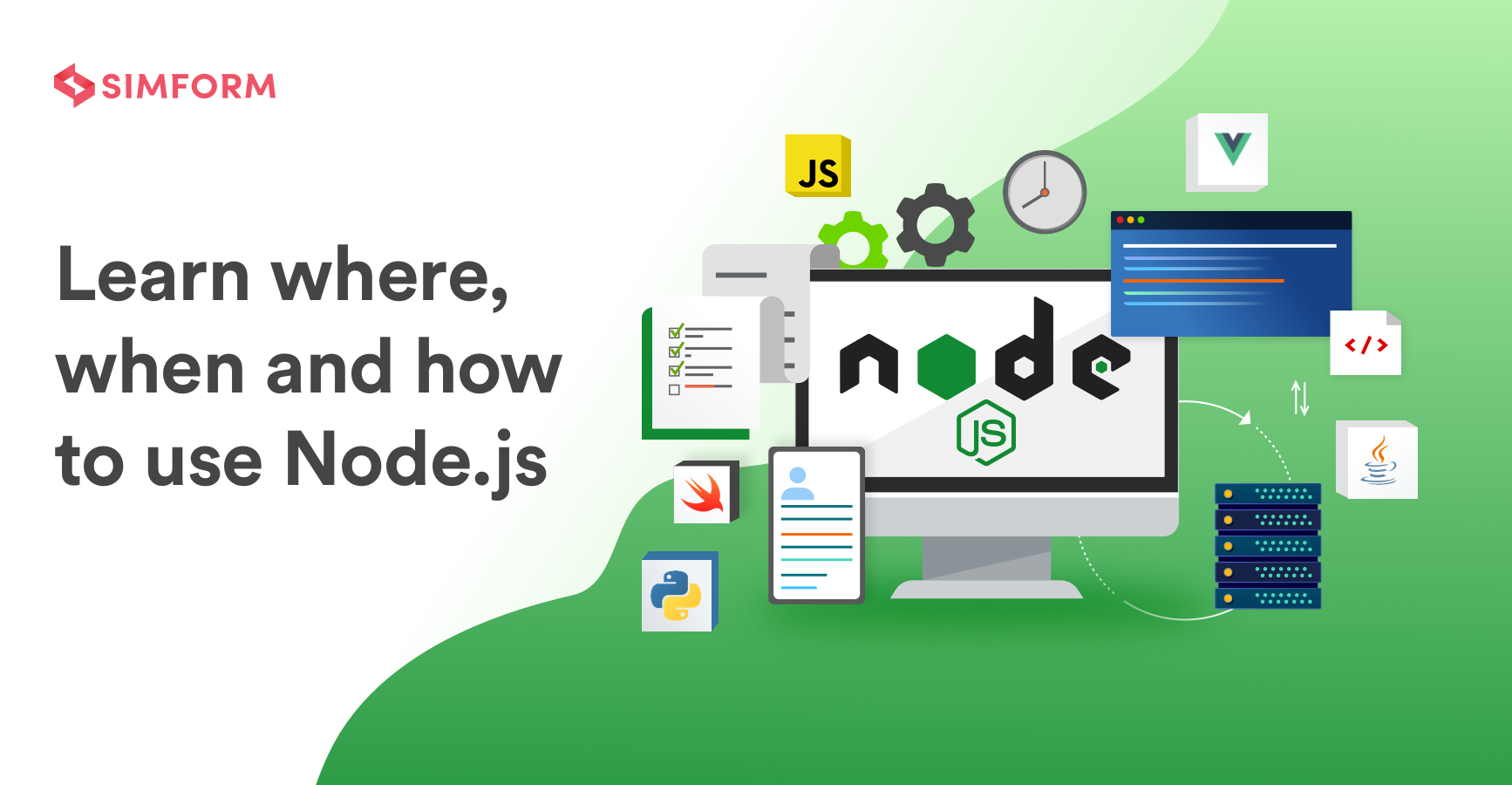
Due to the small size and price of the device, it has become the center of a wide range of projects by tinkerers, makers, and electronics enthusiasts.
Raspberry Pi and Node.js
The Raspberry Pi has a row of GPIO (General Purpose input/output) pins, and these can be used to interact in amazing ways with the real world. This tutorial will focus on how to use these with Node.js.
What Do I Need?
For this tutorial you need a Raspberry Pi. In our examples we use a Raspberry Pi 3, but this tutorial should work for most versions.
Hardware needed:
- Raspberry Pi computer
- MicroSD memory card (We recommend a class 10 with 16 GB or higher)
- MicroSD to SD memory card adapter (usually included with the MicroSD card)
- Micro USB power supply to power the Raspberry Pi (2.5A or greater recommended)
- WiFi/Ethernet Cable connection for the Raspberry Pi (Not needed for Raspberry Pi 3 as it has built in WiFi)
- A working computer with internet and SD memory card reader (used to get the OS (Operating System) for the Raspberry Pi onto the memory card). In our tutorial we use a Windows computer for this, but you can use a Mac or Linux computer if you prefer
- HDMI monitor, USB keyboard (we need these only temporarily for the first boot of the Raspberry Pi)
For later chapters in this tutorial we will use special sensors or devices that we connect to the Raspberry Pi. We will specify these as special requirements in the relevant chapters.
If you already have a Raspberry Pi set up with Raspbian, internet and enabled SSH, you can skip to the step 'Install Node.js on Raspberry Pi'.
Write Raspbian OS Image to MicroSD Card
Before we can start using our Raspberry Pi for anything, we need to get a OS installed.
Raspbian is a free operating system based on Debian Linux, and it is optimized Raspberry Pi.
Download the latest Raspbian image from https://www.raspberrypi.org/downloads/raspbian/ to your computer.
We use the 'LITE' version in our tutorial, since we are setting the Raspberry Pi up as a headless server (we will connect to it through SSH, without having a keyboard/display connected to it). You can use whichever version you want, but this tutorial is written with the 'LITE' version as its focus.
Insert the MicroSD memory card in your computer (via the SD adapter if needed). Open File Explorer to verify that it is operational.
Etcher is a program for flashing images to memory cards. Download and install Etcher from: https://etcher.io/
Launch Etcher:
Click 'Select image' button and find the Raspbian zip file that you downloaded.
Click the 'Select drive' button and specify the memory card as the target location.
Click the 'Flash!' button to write the image to the memory card.
After Etcher is finished writing the image to the memory card, remove it from your computer.
Set up Your Raspberry Pi
C cleaner for mac filehippo. To get the Raspberry Pi ready to boot we need to:
- Insert the MicroSD memory card into the Raspberry Pi
- Connect the USB keyboard
- Connect the HDMI cable
- Connect the USB Wi-Fi adapter (or Ethernet cable). Skip this step if you are using a Raspberry Pi 3
- Connect the micro USB power supply
- The Raspberry Pi should now be booting up
When the Raspberry Pi is finished booting up, log in using username: pi
and password: raspberry
Set Up Network on the Raspberry Pi
If you will use a Ethernet cable to connect your Raspberry Pi to the internet, you can skip this step.
For this section we will assume you have a Raspberry Pi 3, with built in WiFi.
Start by scanning for wireless networks:
This will list all of the available WiFi networks. (It also confirms that your WiFi is working)
Now we need to open the wpa-supplicant file, to add the network you want to connect to:
This will open the file in the Nano editor. Add the following to the bottom of the file (change wifiName
and wifiPassword
with the actual network name and password):
Press 'Ctrl+x
' to save the code. Confirm with 'y
', and confirm the name with 'Enter
'.
And reboot the Raspberry Pi:
After reboot, log in again, and confirm that the WiFi is connected and working:
If the WiFi is working propery, the information displayed should include an IP address, similar to this:
Write down that IP address, as we will use it to connect to the Raspberry Pi via SSH.
Enable SSH, Change Hostname and Password
Now your Raspberry Pi is connected to the internet, it is time to enable SSH.
SSH allows you up use the Raspberry Pi without having a monitor and keyboard connected to it.
(You will need a SSH client for this on your non-Raspberry Pi computer. We use
Open the Raspberry Pi Software Configuration Tool:
You should see a menu like this:
Select option 5 Interfacing Options
:
Select option P2 SSH
, to activate SSH:
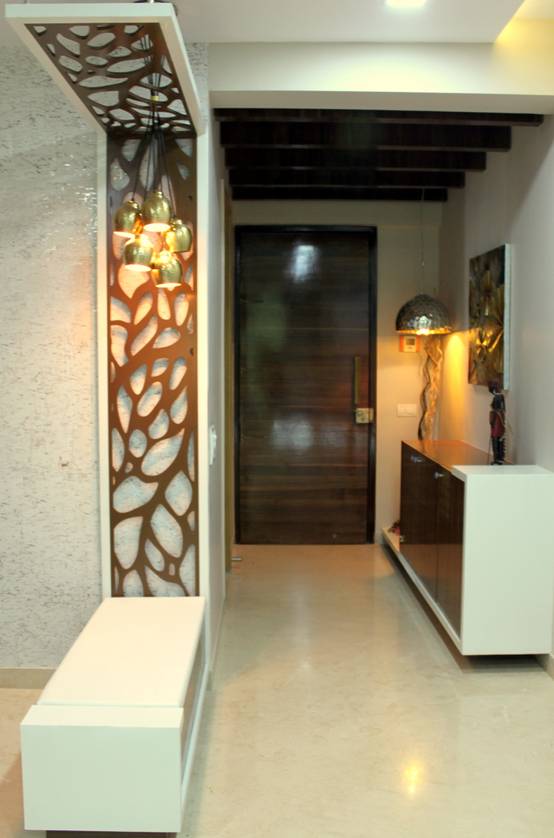
Confirm with YES
, to activate SSH:
SSH is now enabled, and you should be in the main menu again.
Select 1 Change User Password
, and follow the instructions to change the password. Choose a secure password, but something you will remember:
After you have finished changing the password, you should be back in the main menu. Mac mini power supply manual.
Select 2 Hostname
, and follow the instructions to change the hostname:
After you have finished changing the hostname, you should be back in the main menu.
Now we will close the menu and save the changes:
When selecting Finish
, you will get the option to reboot. Select Yes
to reboot the Raspberry Pi.
You can now unplug the monitor and keyboard from the Raspberry Pi, and we can log in using out SSH client.
Open PuTTY, type in the IP address for your Raspberry Pi, and click Open
: Dr cleaner pro mac review.
Log in using the username pi
and the new password you specified.
You should now see a command line like this: (we used w3demopi as our hostname)
You are now able to run your Raspberry Pi in 'Headless-mode', meaning you do not need a monitor or keyboard. And if you have a WiFi connection, you do not need a ethernet cable either, just the power cable!
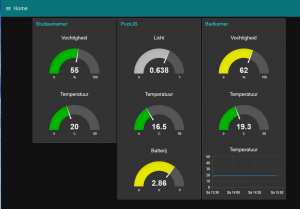
Install Node.js on Raspberry Pi
With the Raspberry Pi properly set up, login in via SSH, and update your Raspberry Pi system packages to their latest versions.
Update your system package list:
Upgrade all your installed packages to their latest version:
Doing this regularly will keep your Raspberry Pi installation up to date.
To download and install newest version of Node.js, use the following command:
Now install it by running:
Check that the installation was successful, and the version number of Node.js with:
Node.js
Get Started with Raspberry Pi and Node.js
Now you have a Raspberry Pi with Node.js installed!
If you want to learn more about Node.js, follow our tutorial: https://www.w3schools.com/nodejs/
In the next chapter we will get to know the GPIO and how to use it with Node.js.
Node.js Mongoose
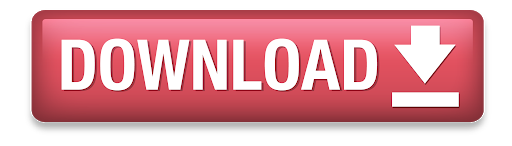